Spring REST - Automate an API Client Request
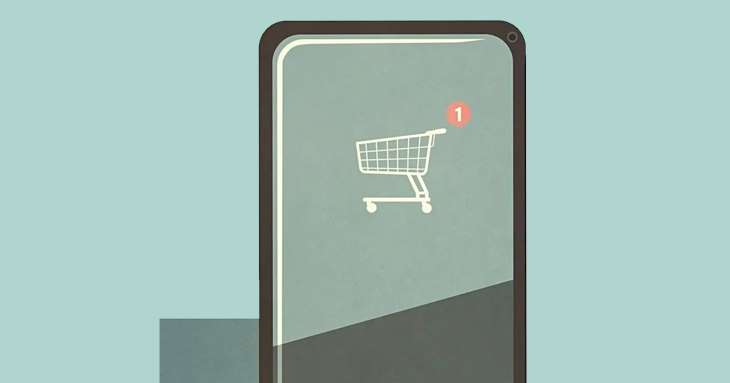
We will create an API Client to automate the creation of an Order based on a combination of Buyers, Suppliers, Customer Numbers, and Articles.
You can find the API specification in the following link.
Test Program
Once a User is logged in, it can access a pool of Buyers. A Buyer can order articles from different Suppliers. But first, every Buyer must be identified by a unique customer number to proceed with creating orders.
We proceed in that way because they are required parameters:
Once the program localize at least on article navigating throuhg these entities, we build an order, send the request and abort the program.
What we need to use in the Program
continue statement
The continue statement is used when we want to skip a particular condition and continue the rest execution. Java continue statement is used for all type of loops but it is generally used in for, while, and do-while loops.
break keyword
The break keyword in Java is used to terminate the execution of a loop or switch statement prematurely. When a break statement is encountered, control is transferred to the statement immediately following the enclosing loop or switch.
OAuth2RestTemplate
Rest template that is able to make OAuth2-authenticated REST requests with the credentials of the provided resource.
We define a boolean variable to control when an article is found.
Mastering API Architecture: Design, Operate, and Evolve Api-Based Systems https://t.co/aMS6zALy4Y via @amazon
— Moises Gamio (@MoisesGamio) November 5, 2024
Here the program code.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
public final class CreateOrderRandomTest {
private static final Logger logger = LoggerFactory.getLogger(CreateOrderRandomTest.class);
private static ResourceOwnerPasswordResourceDetails resourceDetails;
private static OAuth2RestTemplate restTemplate;
private static HttpHeaders headers;
static String apiHost = "http://localhost:41231/cloud";
static String tokenUri = apiHost + "/oauth/token";
static String url = apiHost + "/api/v2/";
static String clientId = "your_clientId";
static String secret = "your_secrret";
public static void main(String[] args) {
headers = new HttpHeaders();
restTemplate = buildRestTemplate(clientId, secret);
try {
String urlRequest = url + "buyers";
HttpEntity<String> entity = new HttpEntity<String>(headers);
ResponseEntity<List<Buyer>> listOfBuyersResponse = restTemplate.exchange(urlRequest,
HttpMethod.GET, entity, new ParameterizedTypeReference<List<Buyer>>(){});
List<Buyer> listOfBuyers = listOfBuyersResponse.getBody();
boolean atLeastOneCustomerNumberWithArticles = false;
for (Buyer buyer : listOfBuyers) {
urlRequest = url + "suppliers";
ResponseEntity<List<Supplier>> listOfSuppliersResponse = restTemplate.exchange(urlRequest + "?buyerId=" + buyer.getBuyerId(),
HttpMethod.GET, entity, new ParameterizedTypeReference<List<Supplier>>(){});
List<Supplier> listOfSuppliers = listOfSuppliersResponse.getBody();
if (listOfSuppliers.size() == 0)
continue;
for (Supplier supplier : listOfSuppliers) {
urlRequest = url + "customerNumbers";
ResponseEntity<List<CustomerNumber>> listOfCustomerNumbersResponse = restTemplate.exchange(
urlRequest + "?buyerId=" + buyer.getBuyerId() + "&supplierId=" + supplier.getSupplierId(),
HttpMethod.GET, entity, new ParameterizedTypeReference<List<CustomerNumber>>(){});
List<CustomerNumber> listOfCustomerNumbers = listOfCustomerNumbersResponse.getBody();
if (listOfCustomerNumbers.size() == 0)
continue;
for (CustomerNumber customerNumber : listOfCustomerNumbers) {
urlRequest = url + "articles";
ResponseEntity<List<Article>> listOfArticlesResponse = restTemplate.exchange(urlRequest + "?buyerId=" + buyer.getBuyerId() +
"&supplierId=" + supplier.getSupplierId() + "&customerNumberId=" + customerNumber.getCustomerNumberId(),
HttpMethod.GET, entity, new ParameterizedTypeReference<List<Article>>(){});
List<Article> listOfArticles = listOfArticlesResponse.getBody();
if (listOfArticles.size() == 0)
continue;
for (Article article : listOfArticles) {
urlRequest = url + "orders";
Order order = buildOrder(buyer, supplier, customerNumber, article);
HttpEntity<Order> orderEntity = new HttpEntity<Order>(order, headers);
ResponseEntity<Order> responseEntity = restTemplate.exchange(urlRequest, HttpMethod.POST, orderEntity, Order.class);
Order orderResponse = responseEntity.getBody();
logger.info(responseEntity.getStatusCode().toString());
break;
}
atLeastOneCustomerNumberWithArticles = true;
logger.info("buyerId = " + buyer.getBuyerId() + ", supplierId = " + supplier.getSupplierId() +
", customerNumberId = " + customerNumber.getCustomerNumberId());
break;
} //end-for listOfCustomerNumbers
if (atLeastOneCustomerNumberWithArticles == true)
break;
} //end-for listOfSuppliers
if (atLeastOneCustomerNumberWithArticles == true)
break;
} //end-for listOfBuyers
} catch (HttpClientErrorException e) {
//code omitted for brevity
} catch (Exception e) {
logger.error("error: " + e.getMessage());
}
}
private static Order buildOrder(Buyer buyer, Supplier supplier, CustomerNumber customerNumber, Article article) {
Order order = new Order();
order.setBuyerId(buyer.getBuyerId());
order.setSupplierId(supplier.getSupplierId());
//code omitted for brevity
return order;
}
private static OAuth2RestTemplate buildRestTemplate(String clientId, String secret) {
resourceDetails = new ResourceOwnerPasswordResourceDetails();
//code omitted for brevity
DefaultOAuth2ClientContext clientContext = new DefaultOAuth2ClientContext();
restTemplate = new OAuth2RestTemplate(resourceDetails, clientContext);
restTemplate.setMessageConverters(asList(new MappingJackson2HttpMessageConverter()));
return restTemplate;
}
}
Please donate to maintain and improve this website if you find this content valuable.