Production-ready Features - Spring Actuator
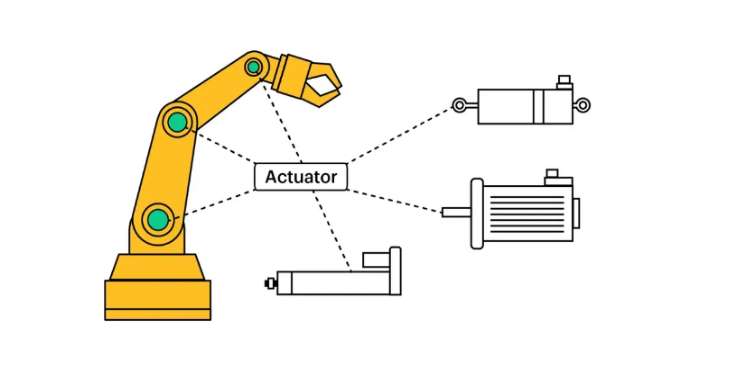
Spring Boot includes additional features to help you monitor and manage your application when deployed to production.
You can manage and monitor your application by using Loggers, Metrics, Tracing, and Auditing automatically.
To enable these Production-ready Features, you need to add a dependency on the spring-boot-starter-actuator starter.
1
2
3
4
5
6
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
</dependencies>
Use case
We want to log all OAuth2 token requests from a new Rest API (successful and unsuccessful).
The authorization grant that the API uses is Resource Owner Password Credentials. See How to implement Spring Boot Security OAuth2.
We are going to enable Auditing to catch authentication and authorization events that the Spring Boot Actuator publishes by default such as “authentication success”, “failure” and “access denied” exceptions.
We define a bean with a listener method.
1
2
3
4
5
6
7
8
9
10
11
12
@Component
public class LoginAttemptsLogger {
private static final Logger logger = LoggerFactory.getLogger(LoginAttemptsLogger.class);
@EventListener
public void auditEventHappened(AuditApplicationEvent auditApplicationEvent) {
logger.info(auditApplicationEvent.getAuditEvent().getPrincipal() + " - " +
auditApplicationEvent.getAuditEvent().getType());
}
}
The previous code works for all endpoints of the API and we will unnecessarily blow up the logfile size.
What we can do, is inject the HttpServletRequest interface that provides request information for HTTP servlets. Then we can validate the oauth/token URL.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
@Component
public class LoginAttemptsLogger {
private static final Logger logger = LoggerFactory.getLogger(LoginAttemptsLogger.class);
@Autowired
private HttpServletRequest httpServletRequest;
@EventListener
public void auditEventHappened(AuditApplicationEvent auditApplicationEvent) {
if (httpServletRequest.getRequestURL().toString().contains("/cloud/oauth/token")) {
logger.info("Login Attempt in /cloud/oauth/token Principal : " +
auditApplicationEvent.getAuditEvent().getPrincipal() + " - " +
auditApplicationEvent.getAuditEvent().getType());
}
}
}
When the authentication process is successful you can see in your console:
1
LoginAttemptsLogger : Login Attempt in /cloud/oauth/token Principal : codersite - AUTHENTICATION_SUCCESS
When the authentication process is not successful you can see in your console:
1
LoginAttemptsLogger : Login Attempt in /cloud/oauth/token Principal : codersite - AUTHENTICATION_FAILURE
See Implementing hot-warm architecture in Elasticsearch to analyze application server logs.
Please support me as a writer. Your donation will help add more articles to this website. Thank you!